By: Team T12-3
Since: Sep 2018
Licence: MIT
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
1.3. Verifying the setup
-
Run the
seedu.address.MainApp
and try a few commands -
Run the tests to ensure they all pass.
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
After forking the repo, the documentation will still have the SE-EDU branding and refer to the se-edu/addressbook-level4
repo.
If you plan to develop this fork as a separate product (i.e. instead of contributing to se-edu/addressbook-level4
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your itemal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
-
Take a look at [GetStartedProgramming].
2. Design
2.1. Architecture
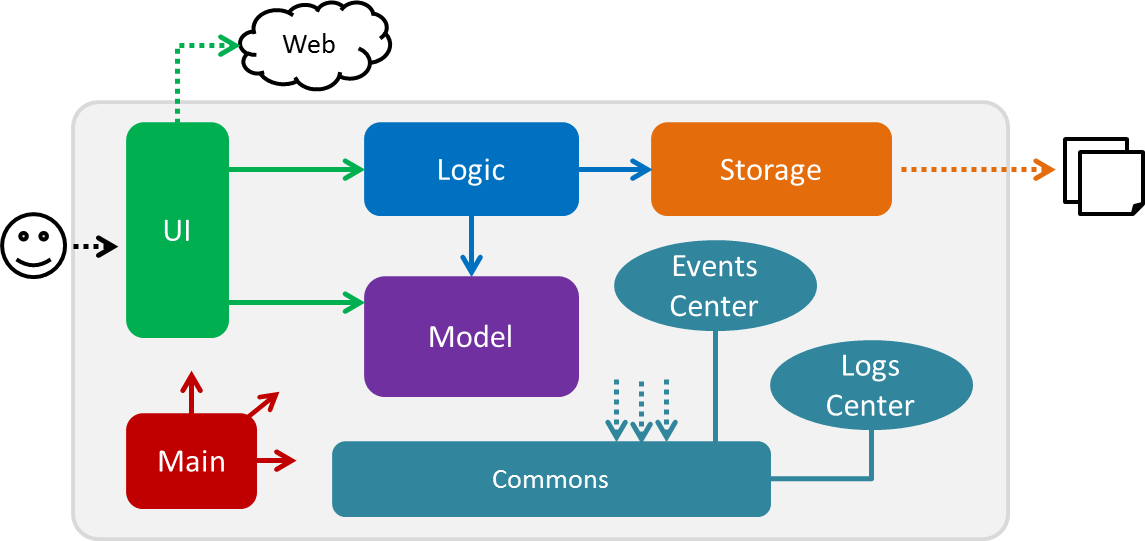
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
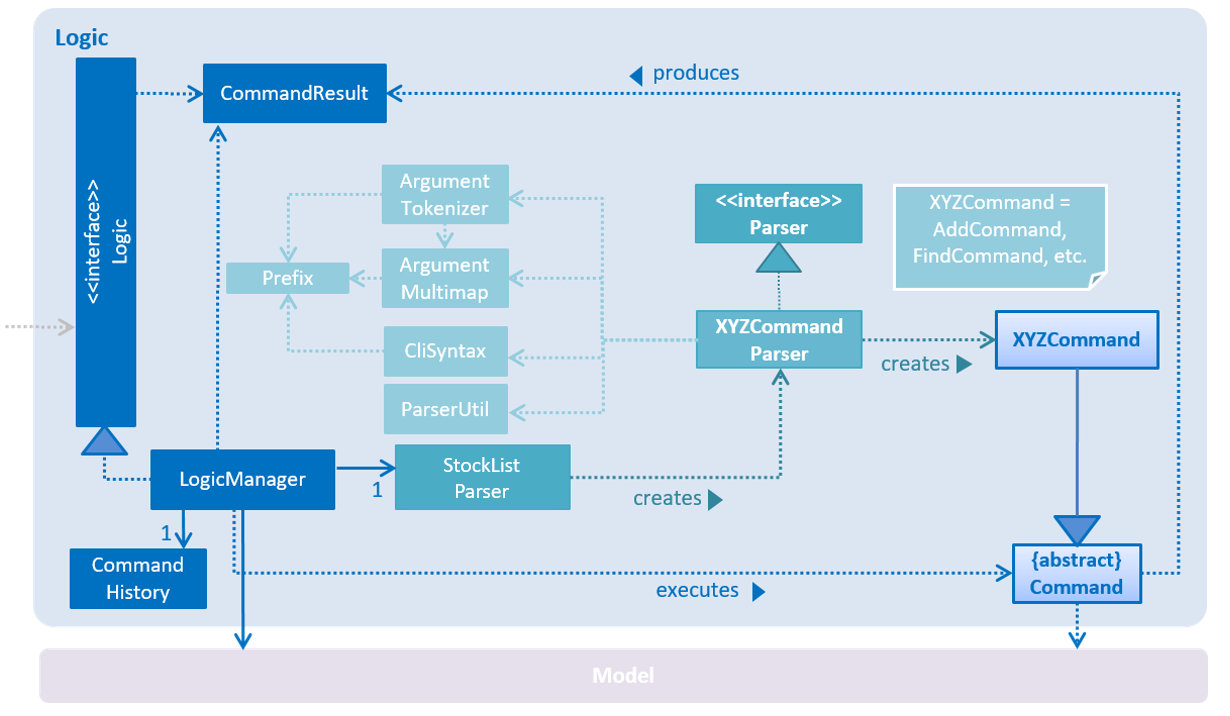
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command delete 1
.
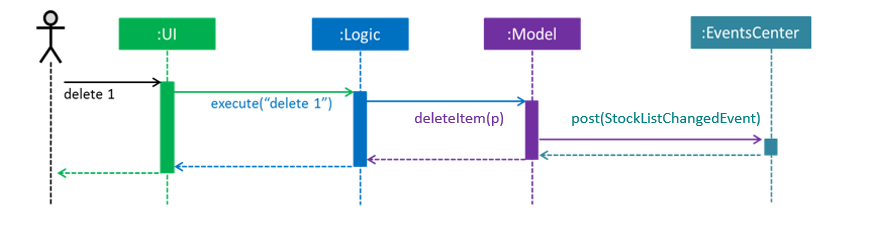
delete 1
command (part 1)
Note how the Model simply raises a StockListChangedEvent when the Stock List data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
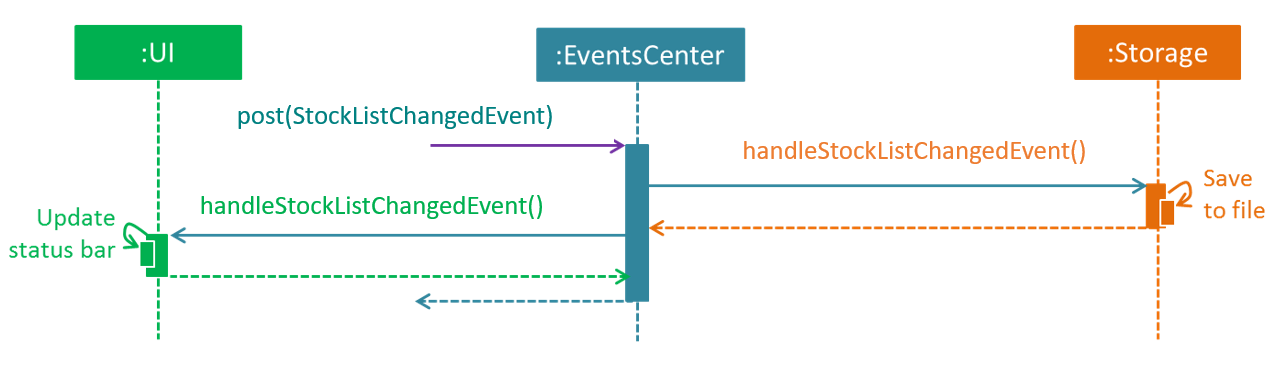
delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
2.2. UI component
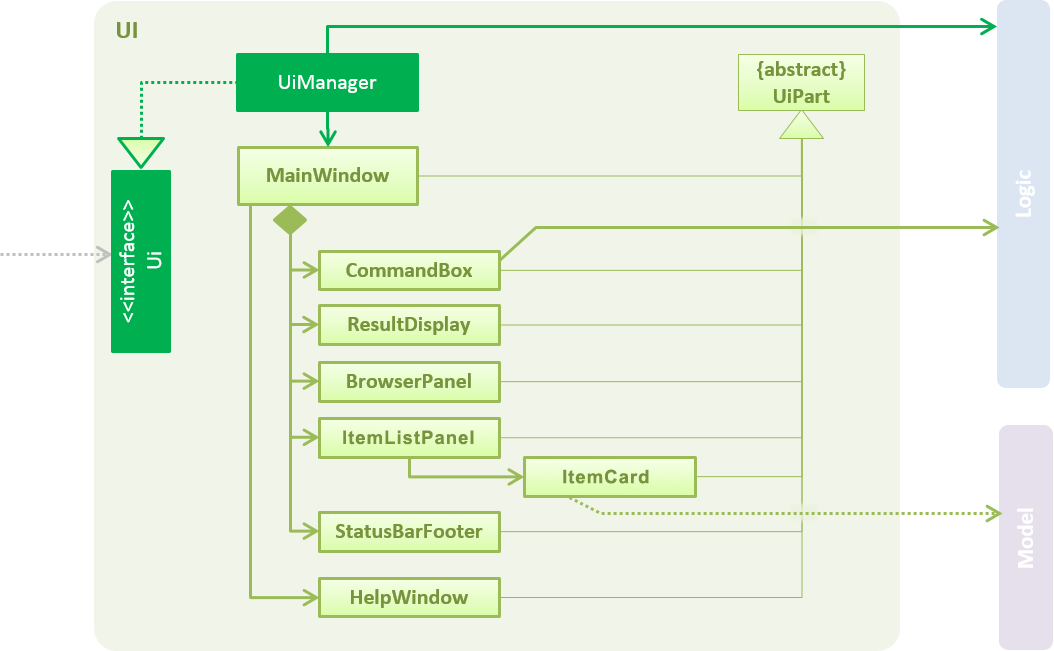
API : Ui.java
The UI consists of a MainWindow
that is made up of parts including CommandBox
, ResultDisplay
, ItemListPanel
, StatusBarFooter
, BrowserPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts is defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
component so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
2.3. Logic component
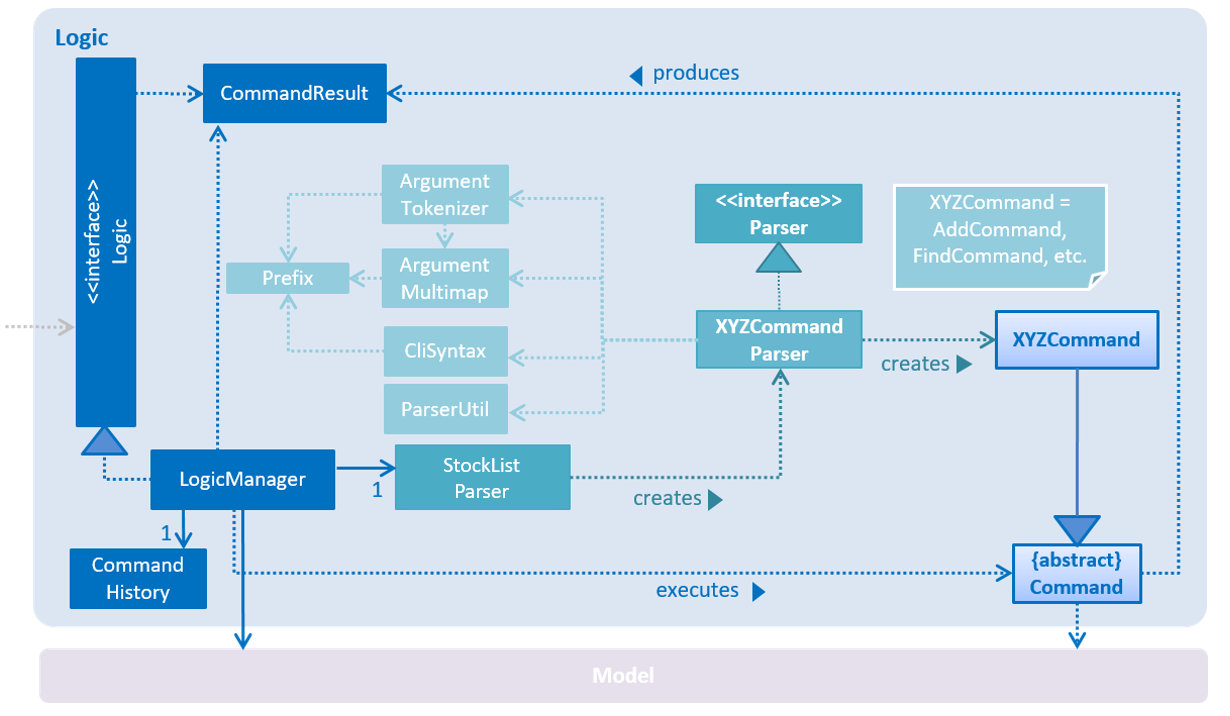
API :
Logic.java
-
Logic
uses theStockListParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a item) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
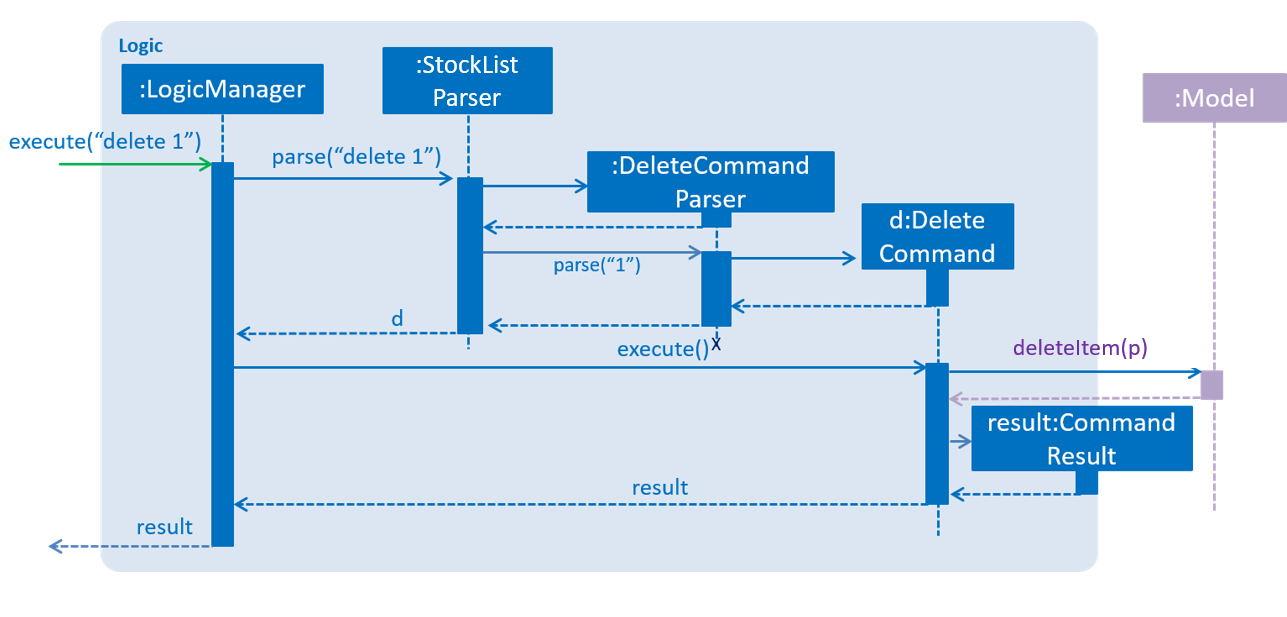
delete 1
Command2.4. Model component
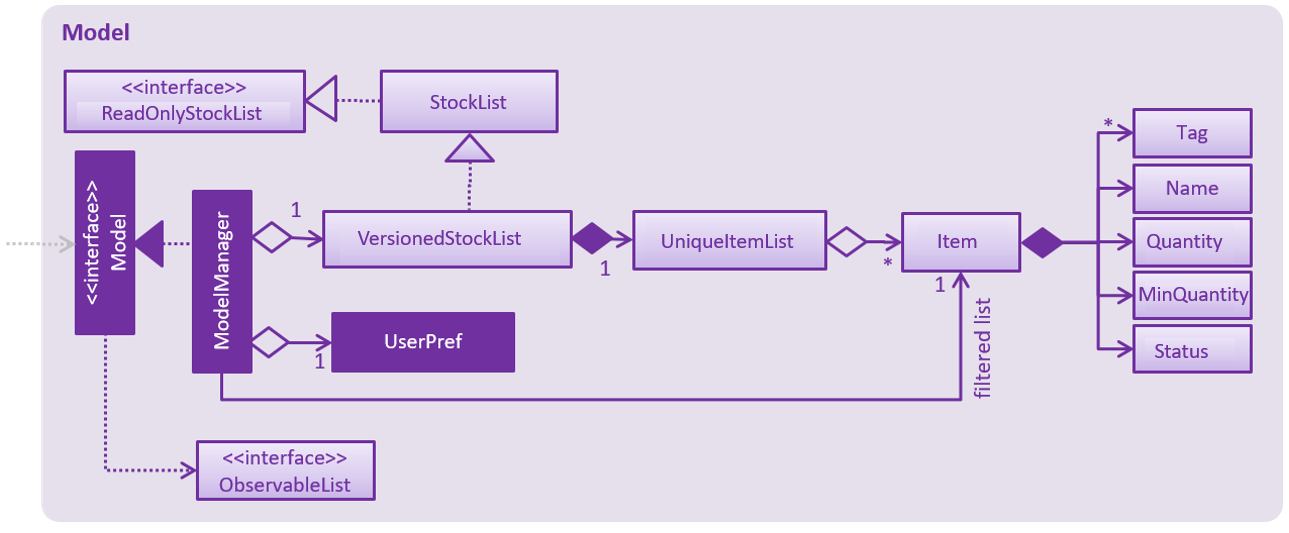
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Stock List data.
-
exposes an unmodifiable
ObservableList<Item>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
As a more OOP model, we can store a Tag list in Stock List , which Item can reference. This would allow Stock List to only require one Tag object per unique Tag , instead of each Item needing their own Tag object. An example of how such a model may look like is given below.![]() |
2.5. Storage component
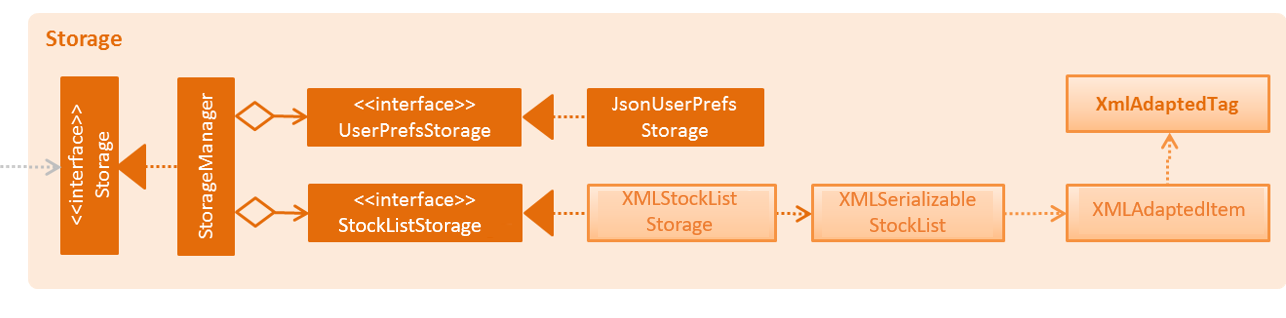
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the Stock List data in xml format and read it back.
2.6. Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Undo/Redo feature
3.1.1. Current Implementation
The undo/redo mechanism is facilitated by VersionedStockList
.
It extends StockList
with an undo/redo history, stored internally as an stockListStateList
and currentStatePointer
.
Additionally, it implements the following operations:
-
VersionedStockList#commit()
— Saves the current stock list state in its history. -
VersionedStockList#undo()
— Restores the previous stock list state from its history. -
VersionedStockList#redo()
— Restores a previously undone stock list state from its history.
These operations are exposed in the Model
interface as Model#commitStockList()
, Model#undoStockList()
and Model#redoStockList()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedStockList
will be initialized with the initial stock list state, and the currentStatePointer
pointing to that single stock list state.

Step 2. The user executes delete 5
command to delete the 5th item in the stock list. The delete
command calls Model#commitStockList()
, causing the modified state of the stock list after the delete 5
command executes to be saved in the stockListStateList
, and the currentStatePointer
is shifted to the newly inserted stock list state.
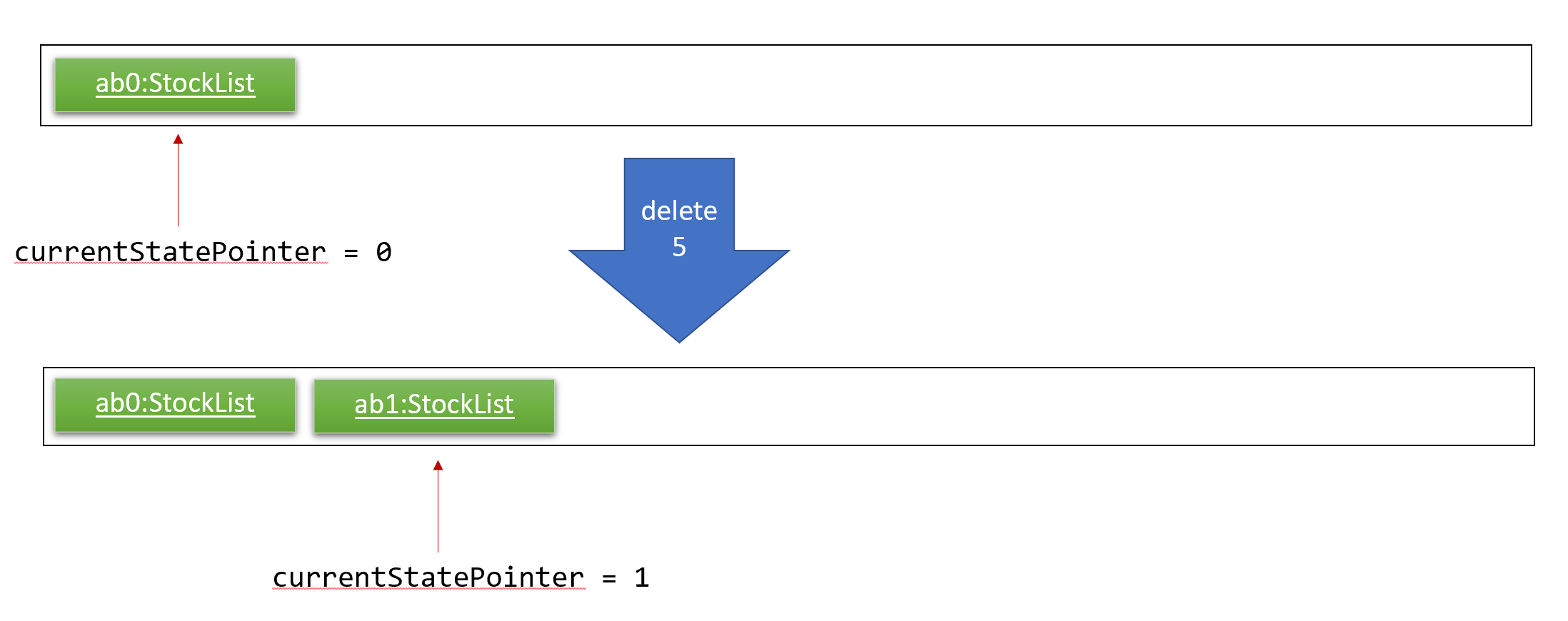
Step 3. The user executes add n/David …
to add a new item. The add
command also calls Model#commitStockList()
, causing another modified stock list state to be saved into the stockListStateList
.
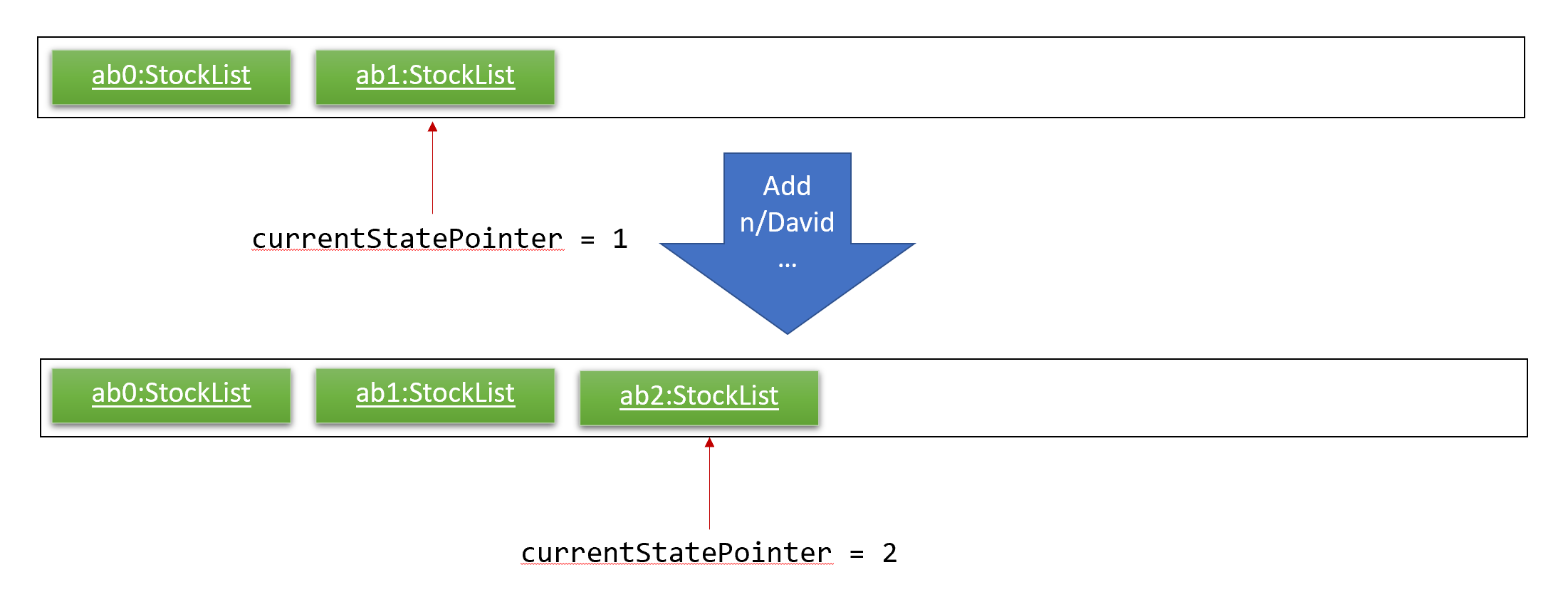
If a command fails its execution, it will not call Model#commitStockList() , so the stock list state will not be saved into the stockListStateList .
|
Step 4. The user now decides that adding the item was a mistake, and decides to undo that action by executing the undo
command. The undo
command will call Model#undoStockList()
, which will shift the currentStatePointer
once to the left, pointing it to the previous stock list state, and restores the stock list to that state.
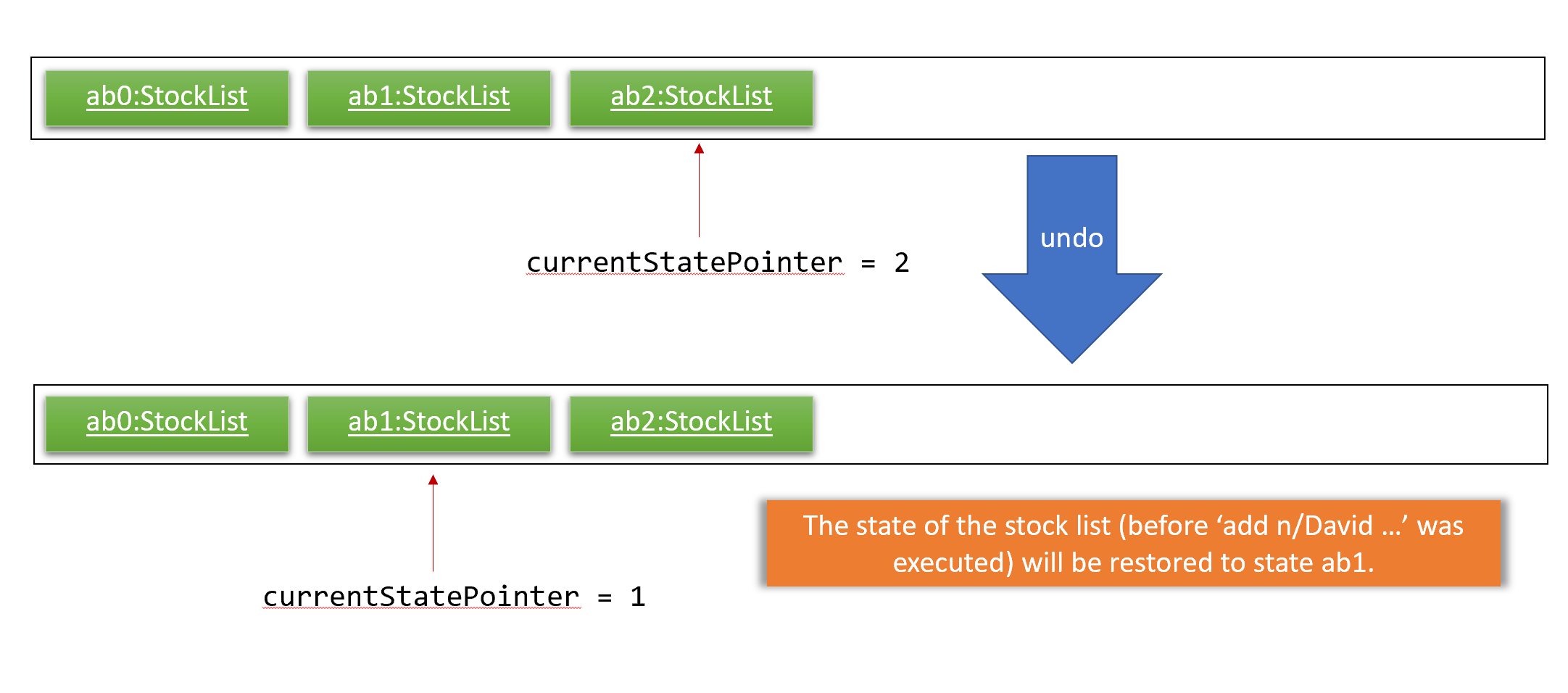
If the currentStatePointer is at index 0, pointing to the initial stock list state, then there are no previous stock list states to restore. The undo command uses Model#canUndoStockList() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the undo.
|
The following sequence diagram shows how the undo operation works:
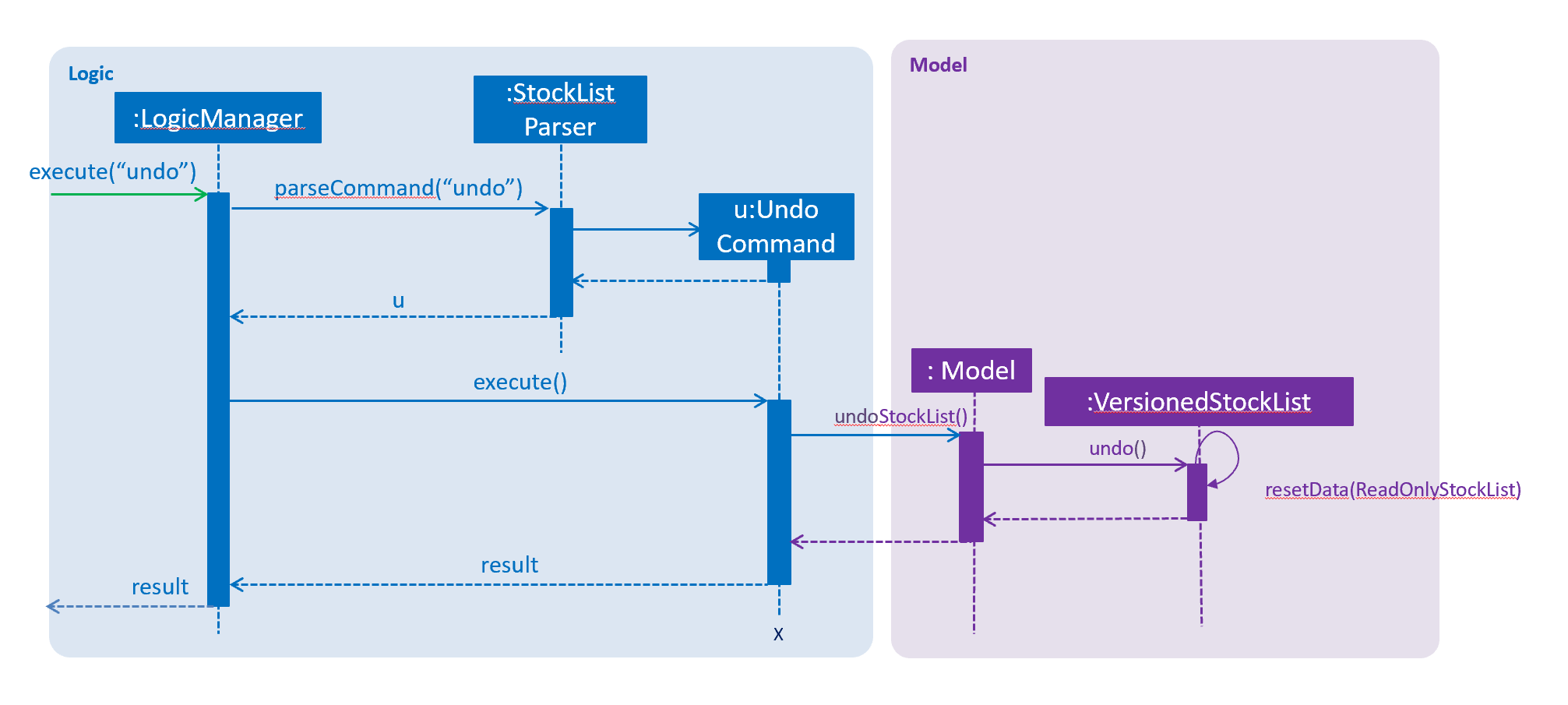
The redo
command does the opposite — it calls Model#redoStockList()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores the stock list to that state.
If the currentStatePointer is at index stockListStateList.size() - 1 , pointing to the latest stock list state, then there are no undone stock list states to restore. The redo command uses Model#canRedoStockList() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
|
Step 5. The user then decides to execute the command list
. Commands that do not modify the stock list, such as list
, will usually not call Model#commitStockList()
, Model#undoStockList()
or Model#redoStockList()
. Thus, the stockListStateList
remains unchanged.
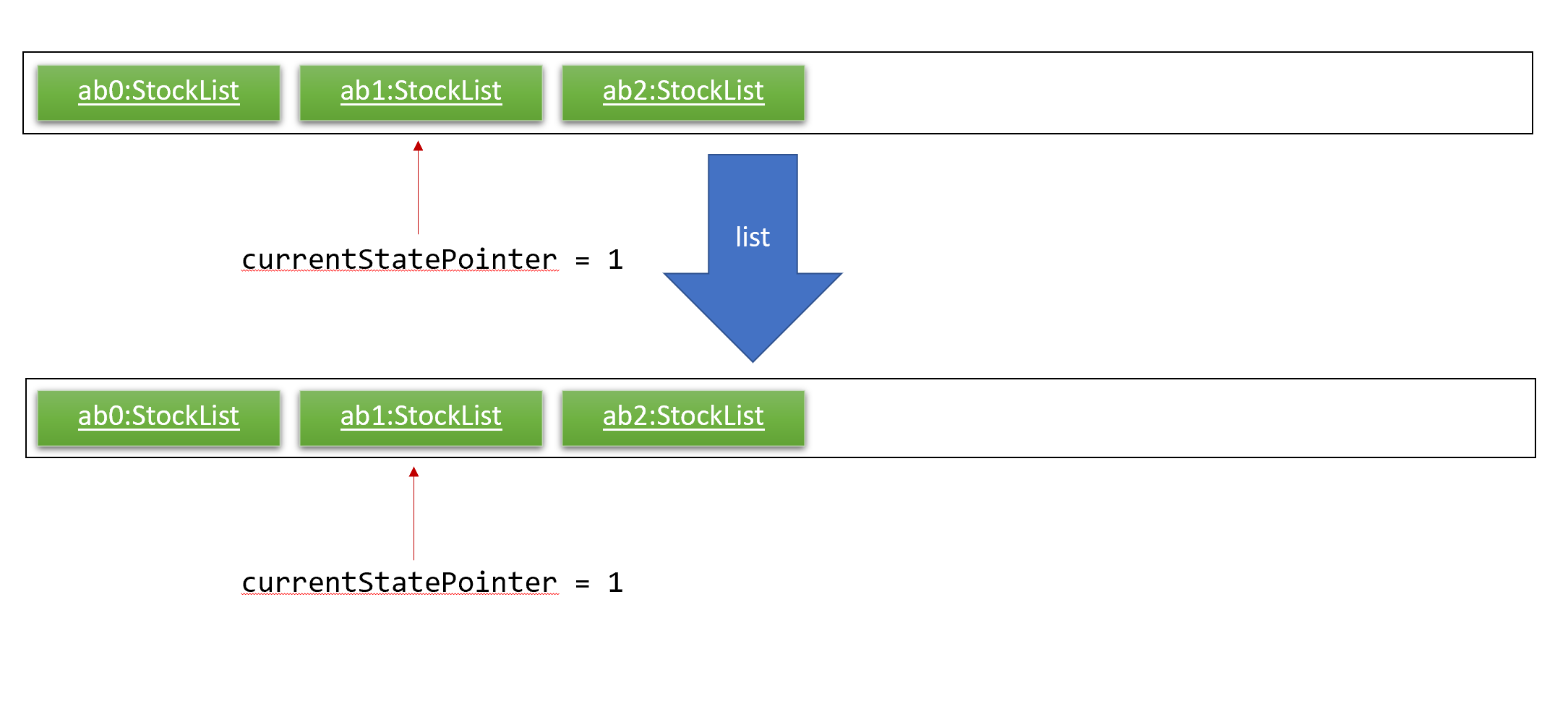
Step 6. The user executes clear
, which calls Model#commitStockList()
. Since the currentStatePointer
is not pointing at the end of the stockListStateList
, all stock list states after the currentStatePointer
will be purged. We designed it this way because it no longer makes sense to redo the add n/David …
command. This is the behavior that most modern desktop applications follow.
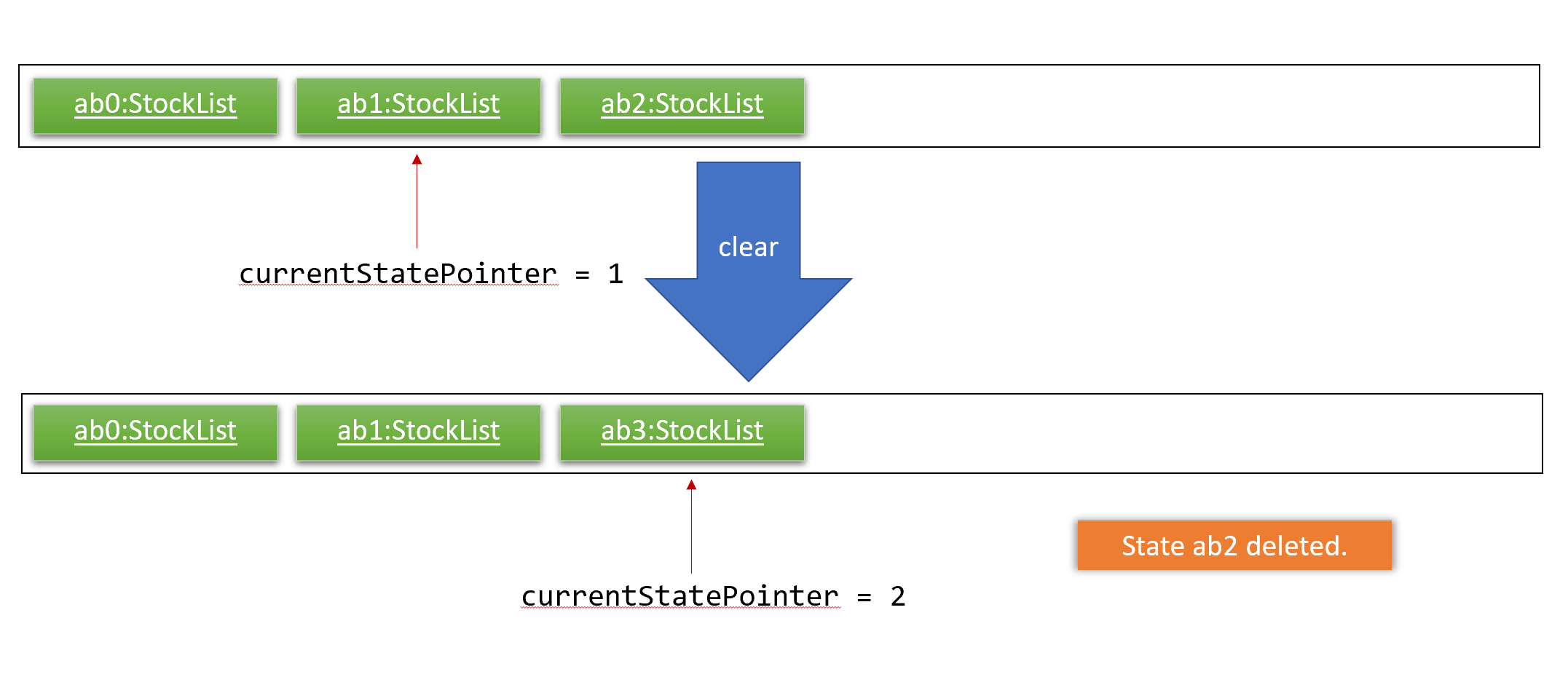
The following activity diagram summarizes what happens when a user executes a new command:
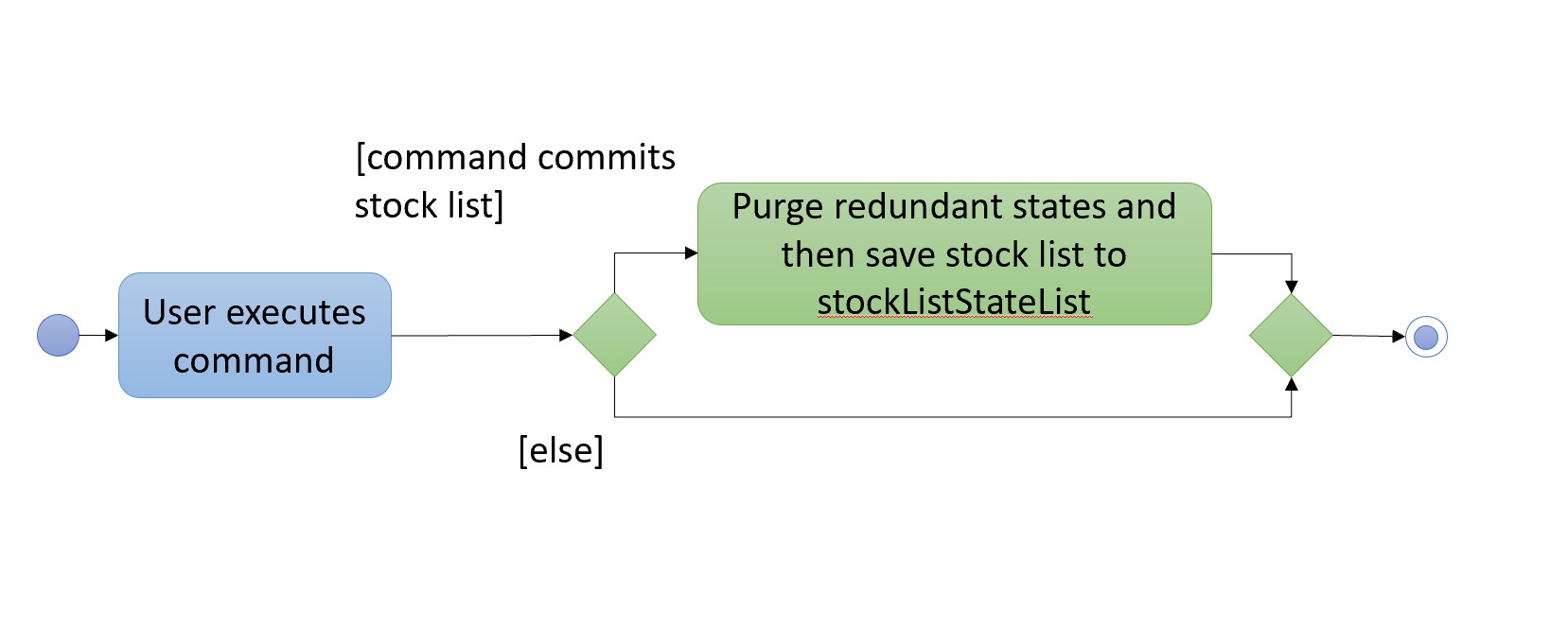
3.1.2. Design Considerations
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire stock list.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
delete
, just save the item being deleted). -
Cons: We must ensure that the implementation of each individual command are correct.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use a list to store the history of stock list states.
-
Pros: Easy for new Computer Science student undergraduates to understand, who are likely to be the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update both
HistoryManager
andVersionedStockList
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate list, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as
HistoryManager
now needs to do two different things.
-
3.2. Save/Open feature
3.2.1. Current Implementation
The save/open mechanism is facilitated by SaveCommand
and OpenCommand
. It extends Command
and implements the following operation:
-
Command#SaveCommand()
— Saves the current version of the stock list as an XML file in a /versions/ folder. -
Command#OpenCommand()
— Opens the saved XML file as a table in the browser panel.
The operation is exposed in the Model
interface as Model#saveStockList()
.
Given below is an example usage scenario and how the saveCommand mechanism behaves at each step.
Step 1. The user executes save april_18
command to save the current version of the stock list as an xml file named april_18.xml
.
Step 2. The save
command calls Model#saveStockList()
, which initiates a saveStockListVersionEvent
. The fileName
and ReadOnlyStockList
are saved as public final variables in the event.
Step 3. The handleSaveStockListVersionEvent
handler calls the saveStockListVersion()
method from the StorageManager
class.
The following sequence diagram shows how the save operation works:
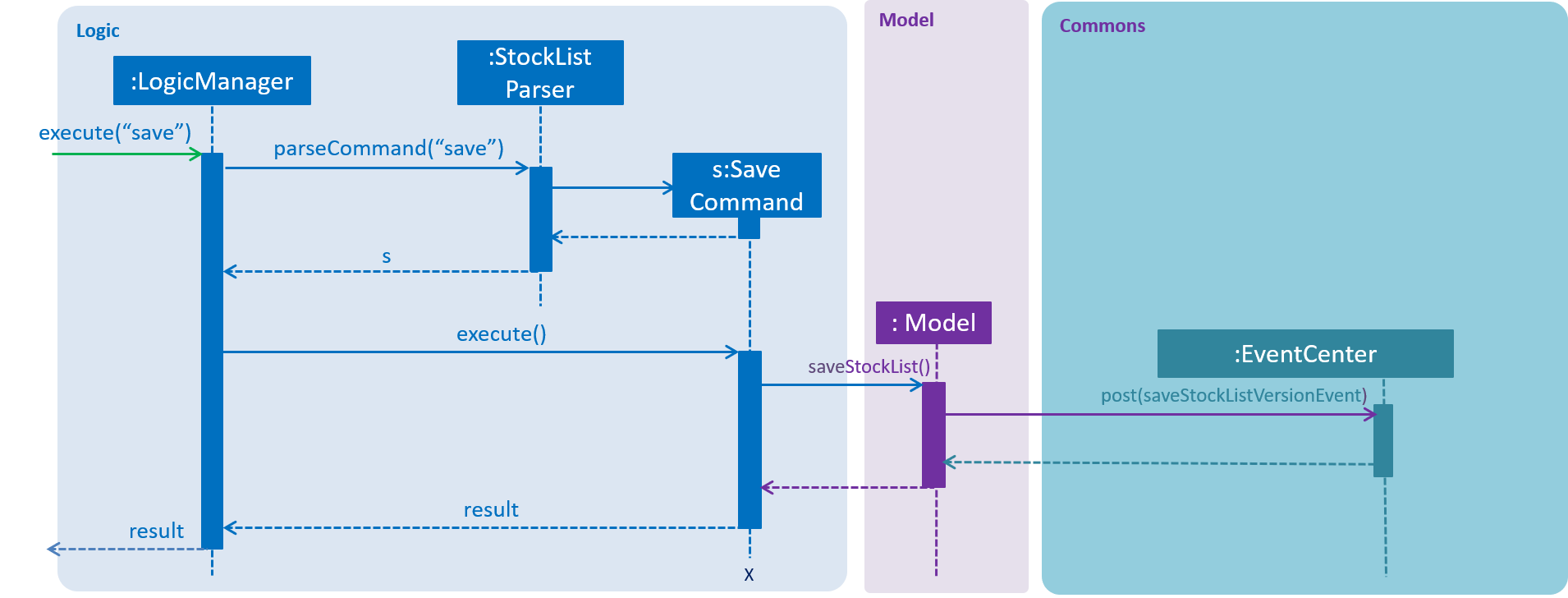
Step 4. The ReadOnlyStockList
is saved as an xml file.
Step 5. The user executes open april_18
command to open the saved april_18.xml file.
Step 6. The open
command calls Model#openStockList()
, which initiates a openStockListVersionEvent
. The fileName
is saved as a public final variable in the event.
Step 7. The handleOpenStockListVersionEvent
handler calls the loadFileAsPage()
method from the BrowserPanel
class.
Step 8. The loadFileAsPage()
method takes in the fileName and passes the directories of the april_18.xml
and template.xsl
files into transformXml()
method.
Step 9. The april_18.xml
file is transformed with the template.xsl
stylesheet into a table format and is displayed by the browser engine on the browser panel.
3.2.2. Design Considerations
Aspect: How save executes
-
Alternative 1 (current choice) Save as .xml file.
-
Pros: Able to display the inventory as a table by using XML transformation with XSLT. The .xml file can also be opened in Excel.
-
Cons: -
-
-
Alternative 2 Save as .csv file.
-
Pros: File can be imported or exported.
-
Cons: Unable to style the displayed table in the application.
-
Aspect: How open executes
-
Alternative 1 (current choice) Transform .xml file into a table with a .xsl stylesheet.
-
Pros: Able to display the inventory in a table format for ease of view.
-
Cons: A template.xsl file must be included in the application.
-
-
Alternative 2 Open the .xml file as it is
-
Pros: Does not require transforming the .xml file with XSLT.
-
Cons: The displayed inventory is in XML format and hard to decipher.
-
3.3. Status feature
3.3.1. Current Implementation
The status feature is facilitated by the Item
class, which contains the Status
class.
-
The status class keeps track of the quantities
Ready
,OnLoan
, andFaulty
.
The status feature is further facilitated by the 5 commands, StatusCommand
, ChangeStatusCommand
, LoanListCommand
, DeleteLoanListCommand
and ViewLoansListCommand
. The commands extend Command
and implements the following operations:
-
Command#StatusCommand()
- Lists out the items according to their status. -
Command#ChangeStatusCommand()
- Changes the status fromReady
toFaulty
, or vice versa. -
Command#LoanListCommand()
- Creates and stores a loan list and changes the item status fromReady
toOnLoan
. -
Command#DeleteLoanListCommand()
- Deletes the loan list and changes the item status fromOnLoan
toReady
. -
Command#ViewLoanListCommand()
- Lists out the loan lists.
Given below is an example usage scenario and how the command mechanisms behaves at each step.
Step 1. The user executes the AddCommand
to add 50 Arduinos into the stock list.
-
The
AddCommand
calls on theItem Constructor
which creates the item object, and sets the status of all 50 Arduinos toReady
by default.
Step 2. The user executes the ChangeStatusCommand
to change the status of 10 Arduinos from Ready
to Faulty
.
-
The
ChangeStatusCommandParser#parseCommand()
is called and takes in the input string from the user, parses it into theChangeStatusDescriptor
, and returns a newChangeStatusCommand
. -
The
ChangeStatusDescriptor
class consists of theitemName
,quantity
,originalStatus
, andupdatedStatus
. -
The
ChangeStatusCommand#execute()
is called. This calls theModel#getFilteredItemList()
to obtain the item to update, and callsModel#updateItem()
to update the item inside of the stock list with the new status.
The following sequence diagram shows how the changeStatus operation works:
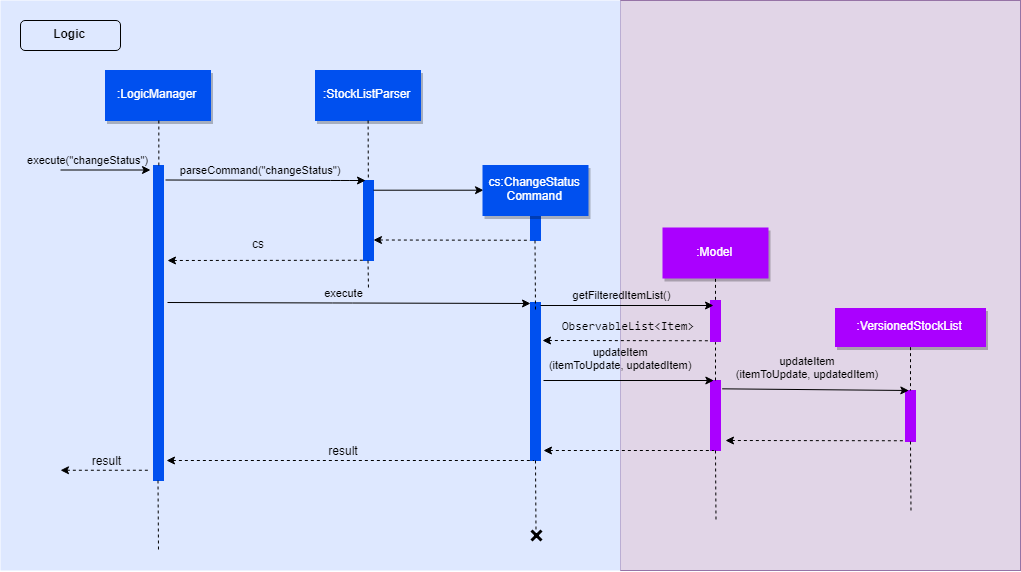
Step 3. The user executes the StatusCommand
to list the items by status.
-
The
StatusCommand#execute
is called. This calls theModel#getFilteredItemList()
to obtain the item list. -
The
StatusCommand#sortSimpleItems()
iterates through the item list and sorts them according to the status. -
The sorted items are stored as
SimpleItem
objects, which stores the justitemName
the andquantity
, as these are the only 2 relevant fields. -
The results are shown to the user.
Step 4. The user executes the LoanListCommand
to loan out 20 Arduinos to Davind.
-
The
LoanListParser#parseCommand
is called and takes in the input string from the user, parses it into theLoanerDescription
, and returns a newLoanListCommand
. -
The
LoanerDescription
class consists of theitemName
,loanerName
, andquantity
. -
The
LoanListCommand#execute()
is called. This creates a newChangeStatusCommand
and calls theChangeStatusCommand#execute()
to change the status from Ready to On_Loan. -
The
LoanListCommand#updateLoanList()
is called. This creates anXmlAdaptedLoanerDescription
object and is added into theXmlAdaptedLoanList
. -
The
LoanListCommand#updateXmlLoanListFile()
is called to save the changes in storage.
Step 5. The user executes the ViewLoanListCommand
to view the loan list.
-
The
XmlAdaptedLoanList#getLoanList()
is used to obtain the loan list -
The
ViewLoanListCommand#getMessageOutput()
iterates through the loan list and returns the result to the user.
Step 6. The user executes the DeleteLoanListCommand
to delete the loan list when Davind returns the Arduinos.
-
The
DeleteLoanListCommand#execute()
is called. This calls theChangeStatusCommand#execute()
to change the status from On_Loan to Ready. -
The
xmlAdaptedLoanList#getLoanList()
is called to obtain the loan list. -
The
ArrayList#remove()
is called to remove the loan list entry -
The
LoanListCommand#updateXmlLoanListFile()
is called to save the changes in storage.
3.3.2. Design Considerations
Aspect: How StatusCommand executes
-
Alternative 1: Storing the items as an Item instead of SimpleItem.
-
Pros: Easier to implement as I would not have to create a new class.
-
Cons: This slows down the code, and it contains redundant information that is not required.
-
-
Alternative 2: Iterate through the item list 3 times, one for each Status field.
-
Pros: We would not have to create 3 ArrayList to store the items as they can be printed out immediately.
-
Cons: This slows down the code significantly, and makes the code longer.
-
Aspect: How ChangeStatusCommand executes
-
Alternative 1: Not creating a ChangeStatusDescriptor class to store the user inputs
-
Pros: Easier to implement as I would not have to create a new class.
-
Cons: This would make the code extremely messy as there would be multiple parameters to handle.
-
3.4. Login feature
3.4.1. Current Implementation
The login mechanism is facilitated by LoginCommand
. It extends Command
and implements the following operations:
-
LoginCommand#modifyLoginStatus()
— checks if the password matches the account in the database, if it exists. If true, updates the logged in account status inModel
accordingly. -
LoginCommand#execute()
— callsLoginCommand#modifyLoginStatus()
. Then, checks login status inModel
and displays a login success message if true and displays a failure message otherwise.
These operations are exposed in the Model
interface as Model#setLoggedInUser()
and Model#getLoginStatus()
respectively.
Given below is an example usage scenario and how the LoginCommand mechanism behaves at each step.
Step 1. The user executes login u/admin p/admin
command to log into StockList with admin and admin being the username and password credentials respectively.
Step 2. The execute
command calls Model#getLoginStatus()
and checks if the user is already logged in. If true, execute
throws a CommandException
notifying the user that he is already logged in.
Step 3. The execute
command then calls LoginCommand#modifyLoginStatus()
, which checks if the username admin exists in the account list, and if it does, checks if the given password admin matches the password associated with the username admin.
Step 4. If the admin password matches, LoginCommand#modifyLoginStatus()
calls Model#setLoggedInUser()
which updates the logged in account status in model
with the logged in account set to admin and logged in status set to true.
Step 5. The execute
command then checks the log in status via Model#getLoginStatus()
. A success message is printed if true; otherwise a failure message is printed.
The following sequence diagram shows how the login operation works:
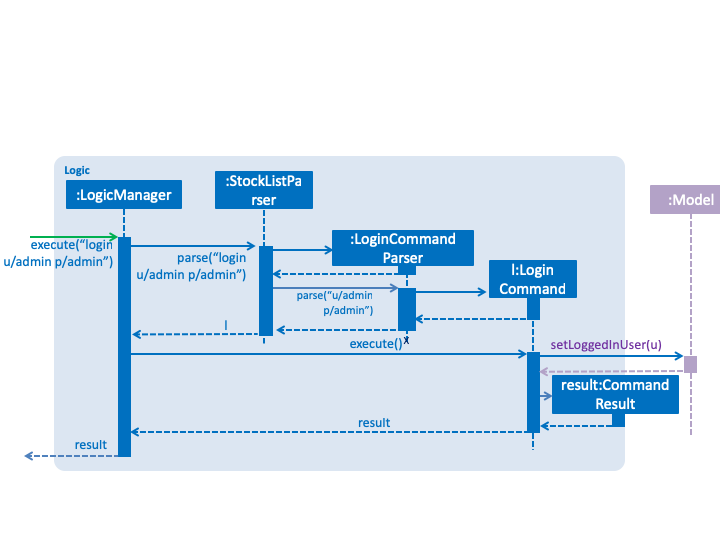
3.4.2. Design Considerations
Aspect: How login executes
-
Alternative 1 (current choice) Check against various accounts stored in a file and allow access if match.
-
Pros: Allows for multiple accounts with access to StockList.
-
Cons: More memory usage.
-
-
Alternative 2 Checks against a single account that can be modified.
-
Pros: Simple to implement, minimal memory usage, allows for only one access account.
-
Cons: Does not allow access for multiple accounts, locked out of app if credentials lost.
-
3.5. Tag feature
3.5.1. Current Implementation
The Tag feature is facilitated by TagCommand
.
It extends Command
and implements the following operation:
-
TagCommand()
— Finds and shows all items under specific tags.
Given below is an example usage scenario and how the tag mechanism behaves at each step.
Step 1. The user executes tag Lab1
command to list all items with the tag Lab1
Step 2. The tag
command calls updateFilteredItemListByTag()
, which shows the search result to the user.
The following sequence diagram shows how the tag operation works:
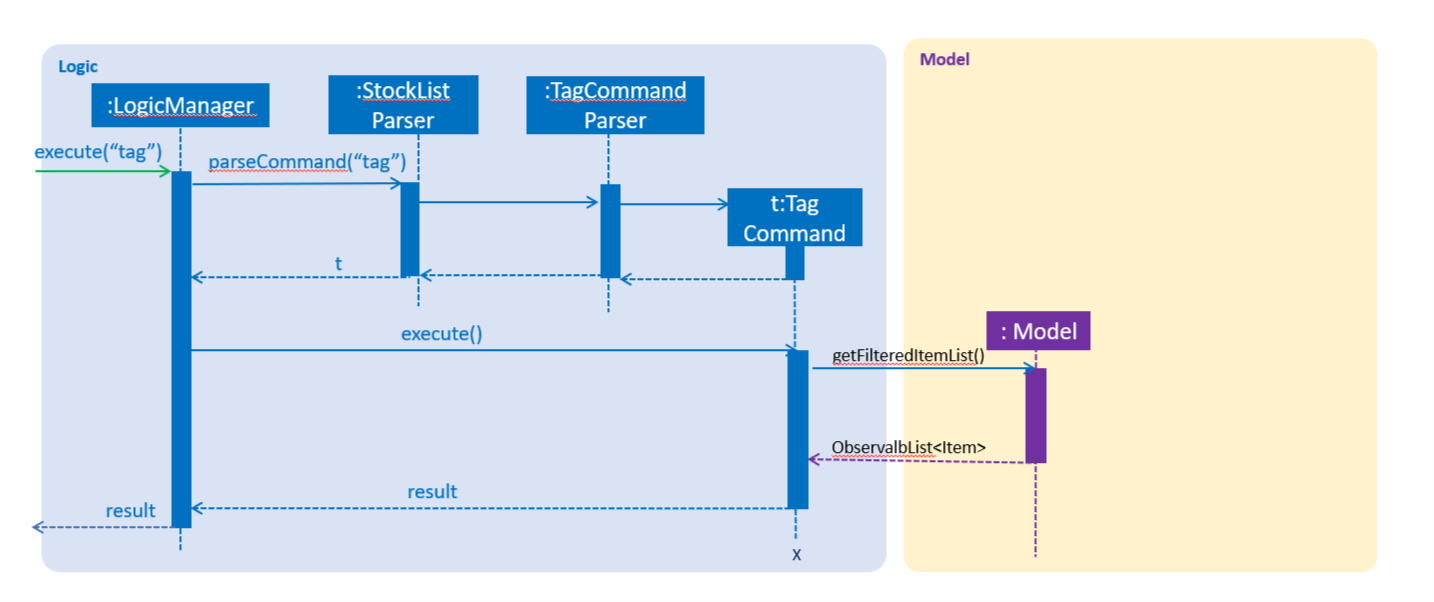
3.5.2. Design Considerations
Aspect: How tagCommand executes
-
Alternative 1 (current choice): When multiple tags are used for search, the search result will be
all the items
contains at least one tag.-
Pros: Easy to implement.
-
Cons: May be difficult for the user to tell which item contains one tag and which items contain the other tag.
-
-
Alternative 2: Group the search result by different tags inputted.
-
Pros: Will be useful in real-life scenario
-
Cons: It is harder to implement and takes up more screen space to show the results.
-
3.6. AddTag/DeleteTag feature
3.6.1. Current Implementation
The AddTag and DeleteTag features are facilitated by AddTagCommand
and DeleteTagCommand
.
They extend Command
and implement the following operations:
-
AddTagCommand()
— Adds new tags to a selected item in the Stock List. -
DeleteTagCommand()
— Deletes some tags while keeping the rest of a selected item in the Stock List.
Given below are example usage scenarios and how the addTag/deleteTag mechanism behave at each step.
Scenario 1: AddTag
Step 1. The user executes addTag 1 t/ Lab2
command to add a tag Lab2
to the item with index 1 in the Stock List.
Step 2. The addTag
command calls updateFilteredItemList();
in model
to show the stock list after the tag is added.
Scenario 2: DeleteTag
Step 1. The user executes deleteTag 1 t/ Lab1
command to delete a tag Lab1
from the item with index 1 in the Stock List.
Step 2. The deleteTag
command calls updateFilteredItemList();
in model
to show the stock list after the tag is deleted.
3.6.2. Design Considerations
-
Alternative 1 (current choice): Adds tags to or deletes tags from one item in the stock list.
-
Pros: Easy to implement.
-
Cons: May be complicated when the same tags need to be added to or deleted from multiple items.
-
-
Alternative 2: Adds tags to or deletes tag from multiple items in the stock list.
-
Pros: Will be more user friendly when same tags need to be added to or deleted from multiple items.
-
Cons: It is harder to implement.
-
3.7. Lost and Found feature
3.7.1. Current Implementation
The Lost and Found mechanism is facilitated by LostCommand
, FoundCommand
and LostandFoundCommand
. These three commands extend Command
. And two class: LostDescriptor and Found Descriptor are created as well. The 3 commands implement the following operations:
* LostCommand#lost()
— Lost an item with its number from the Stock List.
* FoundCommand#found()
— Found a number of lost items from the Stock List.
* LostandFoundCommand#lost&found()
— List the lost items and the lost number.
Given below are example usage scenarios and how the Lost and Found mechanism behaves at each step.
Scenario 1:
Step 1. The user executes lost 1 q/20
command to indicate 20 Arduinos are lost from the Stock List.
Step 2. The LostDescriptor
consists of the lost quantity of the item.
Step 3. The lost
command firstly calls getFilteredItemList()
to get the item of the given index and its original quantity.
Step 4. Then LostDescriptor
will be called and the lost quantity of the item will be returned.
Step 5. By using the original quantity of the item minus the lost quantity, the updated quantity of the item will be got.
Step 6. A copy is created and the change of the quantity is made to the copy. The copy then replaces the original item.
Step 7. The updated item list and success message is shown to the user. Updates are committed to the storage.
Scenario 2:
Step 1. The user executes found 1 q/20
command to indicate 20 lost Arduinos from the Stock List are found.
Step 2. The FoundDescriptor
consists of the found quantity of the item.
Step 3. The found
command firstly calls getFilteredItemList()
to get the item of the given index and its original quantity.
Step 4. Then FoundDescriptor
will be called and the found quantity of the item will be returned.
Step 5. By using the original quantity of the item adds the found quantity, the updated quantity of the item will be got.
Step 6. A copy is created and the change of the quantity is made to the copy. The copy then replaces the original item.
Step 7. The updated item list and success message is shown to the user. Updates are committed to the storage.
Scenario 3:
Step 1. The user executes lost 1 q/5
command to indicate 5 Arduinos are lost.
Step 2. The user executes lost 2 q/3
command to indicate 3 Rasperry Pis are lost.
Step 3. The user executes found 1 q/2
command to indicate 2 lost Arduinos are found.
Step 4. The user executes lost&found
.
Step 5. The lost list will be shown to the user.
3.7.2. Design Considerations
Aspect: How Lost and Found executes
-
Alternative 1 (current choice) Create a lost&found list to record the lost&found history.
-
Pros: Able to list all the lost&found records and history.
-
Cons: May have performance issues in terms of usage and require more memory.
-
-
Alternative 2 When executing lost or found command, update the quantity of the item in the StockList.
-
Pros: Easy to handle while only increasing and decreasing the quantity will be used and no need to record all the lost history.
-
Cons: Unable to list all the lost and found history.
-
3.9. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.10, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.10. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
3.11. Data Encryption (Coming in V2.0)
In v2.0, app data will be encrypted using AES encryption for added security.
4. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
4.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
4.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
4.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
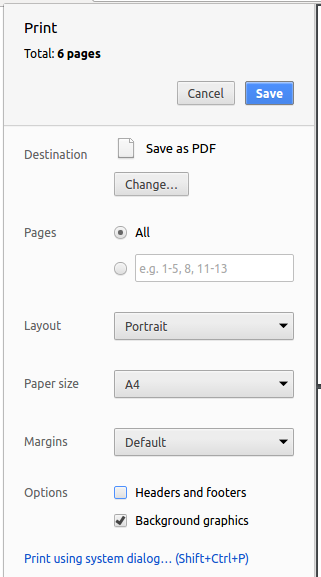
4.4. Site-wide Documentation Settings
The build.gradle
file specifies some project-specific asciidoc attributes which affects how all documentation files within this project are rendered.
Attributes left unset in the build.gradle file will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
The name of the website. If set, the name will be displayed near the top of the page. |
not set |
|
URL to the site’s repository on GitHub. Setting this will add a "View on GitHub" link in the navigation bar. |
not set |
|
Define this attribute if the project is an official SE-EDU project. This will render the SE-EDU navigation bar at the top of the page, and add some SE-EDU-specific navigation items. |
not set |
4.5. Per-file Documentation Settings
Each .adoc
file may also specify some file-specific asciidoc attributes which affects how the file is rendered.
Asciidoctor’s built-in attributes may be specified and used as well.
Attributes left unset in .adoc files will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
Site section that the document belongs to.
This will cause the associated item in the navigation bar to be highlighted.
One of: * Official SE-EDU projects only |
not set |
|
Set this attribute to remove the site navigation bar. |
not set |
4.6. Site Template
The files in docs/stylesheets
are the CSS stylesheets of the site.
You can modify them to change some properties of the site’s design.
The files in docs/templates
controls the rendering of .adoc
files into HTML5.
These template files are written in a mixture of Ruby and Slim.
Modifying the template files in |
5. Testing
5.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
5.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.address.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
5.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
6. Dev Ops
6.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
6.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
6.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
6.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
6.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
6.6. Managing Dependencies
A project often depends on third-party libraries. For example, Stock List depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Product Scope
Target user profile:
-
has a need to manage a significant number of stocks
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: manage stocks faster than a the current method, which is by physical stock taking, or using a spreadsheet.
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
Lab Technician |
Control who has access to my inventory |
Secure my sensitive data |
|
Lab Technician |
Add new items to the list |
Keep track of the new item |
|
Lab Technician |
Update the quantity of an item in the list |
Record the quantity change of the item |
|
Lab Technician |
Update the status of an item in the list |
Record the change in status of the item |
|
Lab Technician |
Delete an item from the list |
Remove all information regarding to the item |
|
Lab Technician |
Show all items |
Check and explore all my items |
|
Lab Technician |
Find a specific item |
Easily check the quantity available |
|
Lab Technician |
List all materials required for a lab session |
Prepare and account for them easily |
|
Lab Technician |
Search for an item with just a partial keyword |
Find items more efficiently |
|
Lab Technician |
Check which items are understocked |
Easily restock them |
|
Lab Technician |
Add one item to use in some labs |
Easily schedule labs |
|
Lab Technician |
Delete one item to use in some labs |
Easily schedule labs and correct my mistakes |
|
Lab Technician |
Record statuses of individual materials (Free, Loan, Faulty) |
Account for the state of items in the lab |
|
Lab Technician |
Report all the lost items |
Record the lost status for all the items and check the lost list whenever I want |
|
Lab Technician |
Withdraw my operation sometime |
Go back to my previous edition anytime |
|
Lab Technician |
Create loan lists for each project group |
Account for items loaned when they are returned |
|
Lab Technician |
Store the quantities of all my items at a given time |
Review them anytime |
{More to be added}
Appendix C: Use Cases:
(For all use cases below, the System is StockList
and the Actor is the user
, unless specified otherwise)
Use case: Delete item
MSS
-
User requests to list items
-
StockList shows a list of items
-
User requests to delete a specific item in the list
-
StockList deletes the item
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. StockList shows an error message.
Use case resumes at step 2.
-
Use case: List items by tag
MSS
-
User requests to list all items
-
StockList shows a list of items
-
User requests to show items with a specific tag
-
StockList finds and shows the items
Use case ends.
Extensions
-
2a. The item list is empty
-
2a1. StockList shows an error message.
Use case ends.
-
-
3a. The given tag is invalid
-
3a1. StockList shows an error message.
Use case resumes at step 2.
-
Use case: Add tags to item
MSS
-
User requests to list all items
-
StockList shows a list of items
-
User requests to add tags to an item
-
Stock list adds the inputted tags to the item
Use case ends.
Extensions
-
2a. The index of item is wrong.
-
2a1. StockList shows an error message.
Use case resumes at step 2.
-
-
3a. The given tag is invalid.
-
3a1. StockList shows an error message.
Use case ends.
-
Use case: Delete some tags of item
MSS
-
User requests to list all items
-
StockList shows a list of items
-
User requests to delete some tags of an item
-
Stock list deletes the inputted tags of the item
Use case ends.
Extensions
-
2a. The index of item is wrong.
-
2a1. StockList shows an error message.
Use case resumes at step 2.
-
-
3a. The given tag does not exist.
-
3a1. StockList shows an error message.
Use case ends.
-
Use case: Lost item
MSS
-
User requests to list items
-
StockList shows a list of items
-
User requests to lose an item with the lost quantity
-
StockList loses the item
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given lost quantity is invalid.
-
3a1. StockList shows an error message.
Use case resumes at step 2.
-
Use case: Found item
MSS
-
User requests to list items
-
StockList shows a list of items
-
User requests to find a lost item with the found quantity
-
StockList found the item
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given found quantity is invalid.
-
3a1. StockList shows an error message.
Use case resumes at step 2.
-
Use case: List lost items
MSS
-
User requests to list lost items
-
StockList shows the list of all lost items
Use case ends.
Use case: Login
MSS
-
User requests to list accounts
-
StockList shows the list of accounts
-
User requests to login using his account credentials
-
StockList shows that the user has successfully logged in
Use case ends.
Extensions
-
2a. The list does not contain user’s account.
Use case ends.
-
3a. The given account credentials are invalid.
-
3a1. StockList shows an error message.
Use case resumes at step 2.
-
Use case: Delete account
MSS
-
User requests to list accounts
-
StockList shows a list of accounts
-
User requests to delete a specific account in the list
-
StockList deletes the account
Use case ends.
Extensions
-
3a. The given index is invalid.
-
3a1. StockList shows an error message.
Use case resumes at step 2.
-
Use case: Add account
MSS
-
User requests to add an account
-
StockList adds the account to the database.
Use case ends.
Extensions
-
1a. The given account is already in the database.
-
1a1. StockList shows an error message.
Use case resumes at step 1.
-
Use case: Edit account
MSS
-
User requests to list accounts
-
StockList shows a list of accounts
-
User requests to edit a specific account in the list
-
StockList edits the account
Use case ends.
Extensions
-
3a. The given index is invalid.
-
3a1. StockList shows an error message.
Use case resumes at step 2.
-
Use case: Save stocklist
MSS
-
User requests to save stocklist
-
StockList saves current inventory as .xml file with specified file name.
Use case ends.
Extensions
-
1a. The file name is invalid.
-
1b. Stocklist shows an error message.
Use case ends.
Use case: Open stocklist version
MSS
-
User requests to open stocklist version
-
StockList opens specified .xml file and displays as table.
Use case ends.
Extensions
-
1a. The file name is invalid.
-
1b. Stocklist shows an error message.
Use case ends.
Use case: List items by status
MSS
-
User requests to list items according to status
-
StockList lists out the item according to the status
Use case ends.
Use case: Change Status
MSS
-
User requests to list all items
-
StockList shows a list the list of all items
-
User requests to change the status of an item.
-
StockList changes the status of the item.
Use case ends.
Extensions
-
3a. Any of the inputs are invalid.
-
3a1. StockList shows an error message.
-
Use case: Creating loan list entry
MSS
-
User requests create a loan list entry
-
StockList creates the loan list entry
-
User requests to view the loan list
-
StockList shows the updated loan list
Use case ends.
Extensions
-
1a. Any of the inputs are invalid.
-
1a1. StockList shows an error message.
-
Use case: Deleting loan list entry
MSS
-
User requests delete a loan list entry
-
StockList deletes the loan list entry
-
User requests to view the loan list
-
StockList shows the updated loan list
Use case ends.
Extensions
-
1a. Any of the inputs are invalid.
-
1a1. StockList shows an error message.
-
Appendix D: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should be able to hold up to 1000 items without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
Appendix E: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
E.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
E.2. Deleting a item
-
Deleting a item while all items are listed
-
Prerequisites: List all items using the
list
command. Multiple items in the list. -
Test case:
delete 1
Expected: First contact is deleted from the list. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No item is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size) {give more}
Expected: Similar to previous.
-
E.3. Lost an item
-
Lost an item while all items are listed
-
Prerequisites: List all items using the
list
command. Multiple items in the list. -
Test case:
lost 1 q/15
Expected: First item is decreased by 15 from the list. Details of the lost item shown in the status message. Timestamp in the status bar is updated. -
Test case:
lost 0 q/15
Expected: No item is lost. Error details shown in the status message. Status bar remains the same. -
Other incorrect lost commands to try:
lost 1 q/x
(where x is larger than the Ready quantity of the first item)
Expected: Similar to previous.
-
E.4. Found a lost item
-
Found a lost item while all items are listed
-
Prerequisites: List all lost items using the
lost&found
command. Multiple items in the list. -
Prerequisites: List all items using the
list
command. Multiple items in the list. -
Test case:
found 1 q/15
Expected: First item is increased by 15 from the list. The corresponding lost quantity of this item will be decreased. Details of the found item shown in the status message. Timestamp in the status bar is updated. -
Test case:
found 0 q/15
Expected: No item is lost. Error details shown in the status message. Status bar remains the same. -
Other incorrect found commands to try:
found 1 q/x
(where x is larger than the lost quantity of the first item)
Expected: Similar to previous.
-
E.5. Logging in
-
Logging in while all accounts are listed
-
Prerequisites: List all accounts using the
listAccounts
command. Only default account 'admin' in the list, with the default credentials. Login status is currently logged out. -
Test case:
login u/admin p/lol
Expected: Account is not logged in. Error details shown in the status message. Status bar remains the same. -
Test case:
login u/admin p/admin
Expected: Account is logged in. Details of the logged in account shown in the status message. Timestamp in the status bar is updated. -
Other incorrect login commands to try:
login
,login u/x p/y
(where x and y differ from the registered account data)
Expected: Similar to the first test case.
-
E.6. Account management
-
Adding an account
-
Prerequisites: List all accounts using the
listAccounts
command. Only default account 'admin' in the list, with the default credentials. Login status is currently logged in as 'admin'. -
Test case:
addAccount u/admin p/lol
Expected: Account is not added. Error details shown in the status message. Status bar remains the same. -
Test case:
addAccount u/john p/doe
Expected: Account is added. Details of the added account shown in the status message. Timestamp in the status bar is updated. -
Other incorrect addAccount commands to try:
addAccount
,addAccount u/x p/y
(where x matches an account already in the database)
Expected: Similar to first test case.
-
-
Editing an account while all accounts are listed
-
Prerequisites: List all accounts using the
listAccounts
command. Accounts 'admin' and 'john' are in the list. Login status is currently logged in as 'admin'. -
Test case:
editAccount 2 u/john p/password123!
Expected: Account is edited. Details of the edited account shown in the status message. Timestamp in the status bar is updated. -
Test case:
editAccount 0 u/chew p/lol
Expected: Account is not edited. Error details shown in the status message. Status bar remains the same. -
Other incorrect editAccount commands to try:
editAccount
,editAccount x u/y p/z
(where x is larger than the account list size)
-
-
Deleting an account while all accounts are listed
-
Prerequisites: List all accounts using the
listAccounts
command. Accounts 'admin' and 'john' are in the list. Login status is currently logged in as 'admin'. -
Test case:
deleteAccount 2
Expected: Account is deleted. Details of the deleted account shown in the status message. Timestamp in the status bar is updated. -
Test case:
deleteAccount 0
Expected: No account is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect deleteAccount commands to try:
deleteAccount
,deleteAccount x
(where x is larger than the account list size)
Expected: Similar to previous.
-
E.7. Saving data
-
Dealing with missing/corrupted data files
-
{explain how to simulate a missing/corrupted file and the expected behavior}
-
{ more test cases … }