Project: Jalil Enterprises Stock List
1. Overview
1.1. Project Overview
This project was a part of the CS2113T (Software Engineering & Object-Oriented Programming) module, and our team was tasked to enhance the existing AddressBook application for a specific target audience group of our choice.
We decided to morph it into Jalil Enterprises, a stock list application for Computer Engineering lab technicians.
This project was inspired by our observations of the work of the lab technician when we were taking our Computer Engineering modules, and ideas on how it could be made more efficient and convenient.
The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java and has ~10 kLoC.
1.2. Project Portfolio Overview
This Project Portfolio is to illustrate the contribution of me, Chew Kin Whye (GitHub: ChewKinWhye) to the JalilEnterprises project.
This includes my feature added, my role in the team, and my contributions to the User Guide and Developer Guide.
2. Features added
For the project, I was responsible for the logic component of the application as well as the implementation of the “status” feature.
The status feature is a vital component of our application. This is be cause as a lab technician, it is not enough to store just the quantity of the items. Additional information regarding the status of the items is required.
-
Code contributed: Project Code Dashboard
-
Major enhancement: : status command.
-
What it does: Allows the user to view the inventory according to the status.
-
Justification: Very often, the lab technician is only concerned with the items of a certain status. For example, he might only be concerned with the items that are Ready.
-
-
Major enhancement: : changeStatus command.
-
What it does: Allows the user change the status of the items.
-
Justification: The status of the items are constantly changing, like when an item becomes faulty. The lab technician needs this command to be able to update the stock list.
-
-
Major enhancement: : loanList, viewLoanList and deleteLoanList command.
-
What it does: Allows the user to manage loan lists when students borrow out items.
-
Justification: The lab technicians are constantly loaning out items to students. Thus, this feature is required to replicate what is done with the previous manual system, and help to digitize it.
-
Highlights: This enhancement required changes in the logic and storage component of the application. Much self directed learning was required to use JAXB and store the data inside an XML file.
-
-
Other contributions:
-
Role as a team leader: For this project, I was assigned to be the team leader, helping to set goals and deadlines, facilitate discussions, allocate tasks among group members, listen to feedback, solve conflicts, boost team morale, and ensure team cohesiveness. Besides that, I contributed significantly to the code and the documentation.
-
Project management:
-
Contribute to the morphing of the application from Address Book to Stock List.
-
Ensuring that the milestones are completed before every deadline.
-
Checking other PRs and features to ensure that the commands are all working and the main code is always functional.
-
Wrote tests for all of my commands implemented.
-
-
3. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
3.1. Listing item according to status: status
Shows the quantity of every item in each status category (Ready, On_Loan, Faulty)
Format: status
3.2. Changing status of item: changeStatus
The status of the items can be changed from Ready to Faulty, and vice-versa
Format: changeStatus n/NAME q/QUANTITY os/ORIGINALSTATUS ns/NEWSTATUS
Examples:
-
changeStatus n/Arduino q/20 os/Ready ns/Faulty
The status of 20 Arduinos would be changed from Ready to Faulty
3.3. Creating a loan list: loanList
A loan list can be created whenever a list of items loaned out needs to be kept tracked on
Format: loanList n/NAME q/QUANTITY l/LOANER
Examples:
-
loanList n/Arduino q/20 l/KinWhye
This would update the status of the 20 arduinos to On_Loan, and add the entry into the loan list
3.4. Viewing the loan list: viewLoanList
Shows every loan list entry
Format: viewLoanList
3.5. Deleting a loan list entry: deleteLoanList
An entry in the loan list can be deleted when the loaner returns the items
Format: deleteLoanList INDEX
Examples:
-
deleteLoanList 1
The first entry as shown on the viewLoanList command will be deleted. The status of the item will be automatically changed back to ready
4. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
4.1. Status feature
4.1.1. Current Implementation
The status feature is facilitated by the Item
class, which contains the Status
class.
-
The status class keeps track of the quantities
Ready
,OnLoan
, andFaulty
.
The status feature is further facilitated by the 5 commands, StatusCommand
, ChangeStatusCommand
, LoanListCommand
, DeleteLoanListCommand
and ViewLoansListCommand
. The commands extend Command
and implements the following operations:
-
Command#StatusCommand()
- Lists out the items according to their status. -
Command#ChangeStatusCommand()
- Changes the status fromReady
toFaulty
, or vice versa. -
Command#LoanListCommand()
- Creates and stores a loan list and changes the item status fromReady
toOnLoan
. -
Command#DeleteLoanListCommand()
- Deletes the loan list and changes the item status fromOnLoan
toReady
. -
Command#ViewLoanListCommand()
- Lists out the loan lists.
Given below is an example usage scenario and how the command mechanisms behaves at each step.
Step 1. The user executes the AddCommand
to add 50 Arduinos into the stock list.
-
The
AddCommand
calls on theItem Constructor
which creates the item object, and sets the status of all 50 Arduinos toReady
by default.
Step 2. The user executes the ChangeStatusCommand
to change the status of 10 Arduinos from Ready
to Faulty
.
-
The
ChangeStatusCommandParser#parseCommand()
is called and takes in the input string from the user, parses it into theChangeStatusDescriptor
, and returns a newChangeStatusCommand
. -
The
ChangeStatusDescriptor
class consists of theitemName
,quantity
,originalStatus
, andupdatedStatus
. -
The
ChangeStatusCommand#execute()
is called. This calls theModel#getFilteredItemList()
to obtain the item to update, and callsModel#updateItem()
to update the item inside of the stock list with the new status.
The following sequence diagram shows how the changeStatus operation works:
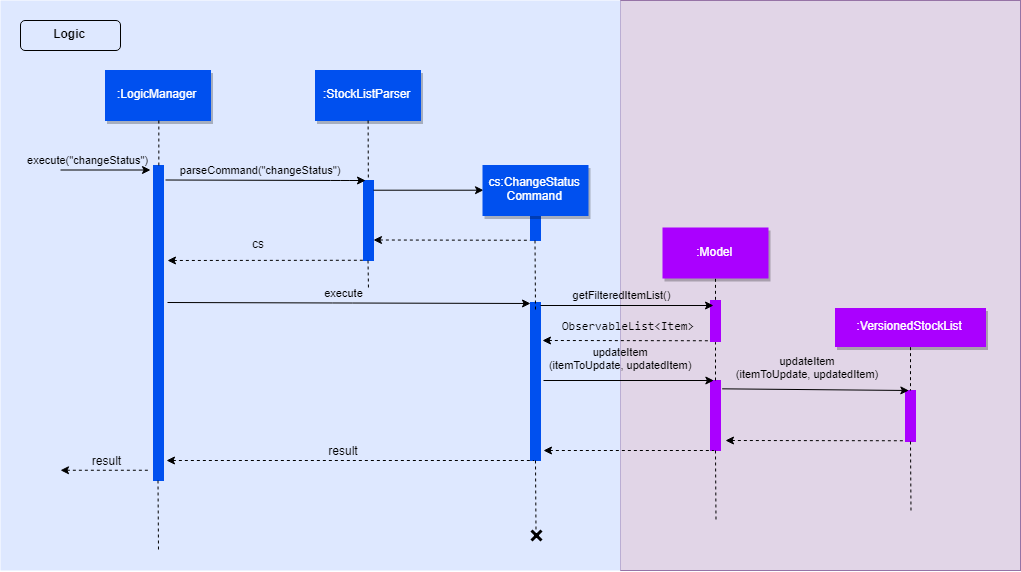
Step 3. The user executes the StatusCommand
to list the items by status.
-
The
StatusCommand#execute
is called. This calls theModel#getFilteredItemList()
to obtain the item list. -
The
StatusCommand#sortSimpleItems()
iterates through the item list and sorts them according to the status. -
The sorted items are stored as
SimpleItem
objects, which stores the justitemName
the andquantity
, as these are the only 2 relevant fields. -
The results are shown to the user.
Step 4. The user executes the LoanListCommand
to loan out 20 Arduinos to Davind.
-
The
LoanListParser#parseCommand
is called and takes in the input string from the user, parses it into theLoanerDescription
, and returns a newLoanListCommand
. -
The
LoanerDescription
class consists of theitemName
,loanerName
, andquantity
. -
The
LoanListCommand#execute()
is called. This creates a newChangeStatusCommand
and calls theChangeStatusCommand#execute()
to change the status from Ready to On_Loan. -
The
LoanListCommand#updateLoanList()
is called. This creates anXmlAdaptedLoanerDescription
object and is added into theXmlAdaptedLoanList
. -
The
LoanListCommand#updateXmlLoanListFile()
is called to save the changes in storage.
Step 5. The user executes the ViewLoanListCommand
to view the loan list.
-
The
XmlAdaptedLoanList#getLoanList()
is used to obtain the loan list -
The
ViewLoanListCommand#getMessageOutput()
iterates through the loan list and returns the result to the user.
Step 6. The user executes the DeleteLoanListCommand
to delete the loan list when Davind returns the Arduinos.
-
The
DeleteLoanListCommand#execute()
is called. This calls theChangeStatusCommand#execute()
to change the status from On_Loan to Ready. -
The
xmlAdaptedLoanList#getLoanList()
is called to obtain the loan list. -
The
ArrayList#remove()
is called to remove the loan list entry -
The
LoanListCommand#updateXmlLoanListFile()
is called to save the changes in storage.
4.1.2. Design Considerations
Aspect: How StatusCommand executes
-
Alternative 1: Storing the items as an Item instead of SimpleItem.
-
Pros: Easier to implement as I would not have to create a new class.
-
Cons: This slows down the code, and it contains redundant information that is not required.
-
-
Alternative 2: Iterate through the item list 3 times, one for each Status field.
-
Pros: We would not have to create 3 ArrayList to store the items as they can be printed out immediately.
-
Cons: This slows down the code significantly, and makes the code longer.
-
Aspect: How ChangeStatusCommand executes
-
Alternative 1: Not creating a ChangeStatusDescriptor class to store the user inputs
-
Pros: Easier to implement as I would not have to create a new class.
-
Cons: This would make the code extremely messy as there would be multiple parameters to handle.
-